NLTK
NLTK is a powerful open-source Python toolkit for text analysis and natural language processing, offering extensive features for academic and industrial applications.
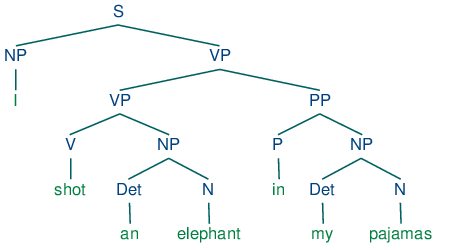
NLTK
NLTK is a comprehensive Python toolkit for symbolic and statistical NLP, offering features like tokenization, stemming, lemmatization, POS tagging, and more. It’s widely used in academia and industry for text analysis and language processing tasks.
Natural Language Toolkit (NLTK) is a comprehensive suite of libraries and programs designed for symbolic and statistical natural language processing bridges human-computer interaction. Discover its key aspects, workings, and applications today!") (NLP) for the Python programming language. Developed initially by Steven Bird and Edward Loper, NLTK is a free, open-source project that is widely used in both academic and industrial settings for text analysis and language processing. It is particularly noted for its ease of use and extensive collection of resources, including over 50 corpora and lexical resources. NLTK supports a variety of NLP tasks, such as tokenization, stemming, tagging, parsing, and semantic reasoning, making it a versatile tool for linguists, engineers, educators, and researchers alike.
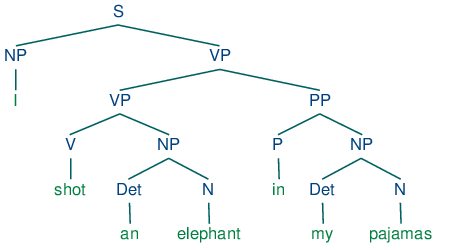
Key Features and Capabilities
Tokenization
Tokenization is the process of breaking down text into smaller units such as words or sentences. In NLTK, tokenization can be performed using functions like word_tokenize
and sent_tokenize
, which are essential for preparing text data for further analysis. The toolkit provides easy-to-use interfaces for these tasks, allowing users to efficiently preprocess text data.
Example:
from nltk.tokenize import word_tokenize, sent_tokenize
text = "NLTK is a great tool. It is widely used in NLP."
word_tokens = word_tokenize(text)
sentence_tokens = sent_tokenize(text)
Stop Words Removal
Stop words are common words that are often removed from text data to reduce noise and focus on meaningful content. NLTK provides a list of stop words for various languages, aiding in tasks like frequency analysis and sentiment analysis. This functionality is crucial for improving the accuracy of text analysis by filtering out irrelevant words.
Example:
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in word_tokens if word.lower() not in stop_words]
Stemming
Stemming involves reducing words to their root form, often by removing prefixes or suffixes. NLTK offers several stemming algorithms, such as the Porter Stemmer, which is commonly used to simplify words for analysis. Stemming is particularly useful in applications where the exact word form is less important than its root meaning.
Example:
from nltk.stem import PorterStemmer
stemmer = PorterStemmer()
stems = [stemmer.stem(word) for word in word_tokens]
Lemmatization
Lemmatization is similar to stemming but results in words that are linguistically correct, often using a dictionary to determine the root form of a word. NLTK’s WordNetLemmatizer
is a popular tool for this purpose, allowing for more accurate text normalization.
Example:
from nltk.stem import WordNetLemmatizer
lemmatizer = WordNetLemmatizer()
lemmas = [lemmatizer.lemmatize(word) for word in word_tokens]
Part-of-Speech (POS) Tagging
POS Tagging assigns parts of speech to each word in a text, such as noun, verb, adjective, etc., which is crucial for understanding the syntactic structure of sentences. NLTK’s pos_tag
function facilitates this process, enabling more detailed linguistic analysis.
Example:
import nltk
pos_tags = nltk.pos_tag(word_tokens)
Named Entity Recognition (NER)
Named Entity Recognition identifies and categorizes key entities in text, such as names of people, organizations, and locations. NLTK provides functions to perform NER: a key AI tool in NLP for identifying and classifying entities in text, enhancing data analysis."), enabling more advanced text analysis that can extract meaningful insights from documents.
Example:
from nltk import ne_chunk
entities = ne_chunk(pos_tags)
Frequency Distribution
Frequency Distribution is used to determine the most common words or phrases within a text. NLTK’s FreqDist
function helps in visualizing and analyzing word frequencies, which is fundamental for tasks like keyword extraction and topic modeling.
Example:
from nltk import FreqDist
freq_dist = FreqDist(word_tokens)
Parsing and Syntax Tree Generation
Parsing involves analyzing the grammatical structure of sentences. NLTK can generate syntax trees, which represent the syntactic structure, aiding in deeper linguistic analysis. This is essential for applications like machine translation and syntactic parsing.
Example:
from nltk import CFG
from nltk.parse.generate import generate
grammar = CFG.fromstring("""
S -> NP VP
NP -> 'NLTK'
VP -> 'is' 'a' 'tool'
""")
parser = nltk.ChartParser(grammar)
Text Corpora
NLTK includes access to a variety of text corpora, which are essential for training and evaluating NLP models. These resources can be easily accessed and utilized for various processing tasks, providing a rich dataset for linguistic research and application development.
Example:
from nltk.corpus import gutenberg
sample_text = gutenberg.raw('austen-emma.txt')
Use Cases and Applications
Academic Research
NLTK is widely used in academic research for teaching and experimenting with natural language processing concepts. Its extensive documentation and resources make it a preferred choice for educators and students. NLTK’s community-driven development ensures that it remains up-to-date with the latest advancements in NLP.
Text Processing and Analysis
For tasks such as sentiment analysis, topic modeling, and information extraction, NLTK provides an array of tools that can be integrated into larger systems for text processing. These capabilities make it a valuable asset for businesses looking to leverage text data for insights.
Machine Learning Integration
NLTK can be combined with machine learning libraries like scikit-learn and TensorFlow to build more intelligent systems that understand and process human language. This integration allows for the development of sophisticated NLP applications, such as chatbots and AI-driven systems.
Computational Linguistics
Researchers in computational linguistics use NLTK to study and model linguistic phenomena, leveraging its comprehensive toolkit to analyze and interpret language data. NLTK’s support for multiple languages makes it a versatile tool for cross-linguistic studies.
Installation and Setup
NLTK can be installed via pip, and additional datasets can be downloaded using the nltk.download()
function. It supports multiple platforms, including Windows, macOS, and Linux, and requires Python 3.7 or later. Installing NLTK in a virtual environment is recommended to manage dependencies efficiently.
Installation Command:
pip install nltk
Research
NLTK: The Natural Language Toolkit (Published: 2002-05-17)
This foundational paper by Edward Loper and Steven Bird introduces NLTK as a comprehensive suite of open-source modules, tutorials, and problem sets aimed at computational linguistics. NLTK covers a broad spectrum of natural language processing tasks, both symbolic and statistical, and provides an interface to annotated corpora. The toolkit is designed to facilitate learning through hands-on experience, allowing users to manipulate sophisticated models and learn structured programming. Read moreText Normalization for Low-Resource Languages of Africa (Published: 2021-03-29)
This study explores the application of NLTK in text normalization and language model training for low-resource African languages. The paper highlights the challenges faced in machine learning when dealing with data of dubious quality and limited availability. By utilizing NLTK, the authors developed a text normalizer using the Pynini framework, demonstrating its effectiveness in handling multiple African languages, thereby showcasing NLTK’s versatility in diverse linguistic environments. Read moreNatural Language Processing, Sentiment Analysis and Clinical Analytics (Published: 2019-02-02)
This paper examines the intersection of NLP, sentiment analysis, and clinical analytics, emphasizing the utility of NLTK. It discusses how advancements in big data have enabled healthcare professionals to extract sentiment and emotion from social media data. NLTK is highlighted as a crucial tool in implementing various NLP theories, facilitating the extraction and analysis of valuable insights from textual data, thereby enhancing clinical decision-making processes. Read more
Frequently asked questions
- What is NLTK?
NLTK (Natural Language Toolkit) is a comprehensive suite of Python libraries and programs for symbolic and statistical natural language processing (NLP). It offers tools for tokenization, stemming, lemmatization, POS tagging, parsing, and more, making it widely used in both academic and industrial settings.
- What can you do with NLTK?
With NLTK, you can perform a wide range of NLP tasks, including tokenization, stop words removal, stemming, lemmatization, part-of-speech tagging, named entity recognition, frequency distribution analysis, parsing, and working with text corpora.
- Who uses NLTK?
NLTK is used by researchers, engineers, educators, and students in academia and industry for building NLP applications, experimenting with language processing concepts, and teaching computational linguistics.
- How do you install NLTK?
You can install NLTK using pip with the command 'pip install nltk'. Additional datasets and resources can be downloaded within Python using 'nltk.download()'.
- Can NLTK be integrated with machine learning libraries?
Yes, NLTK can be integrated with machine learning libraries such as scikit-learn and TensorFlow to build advanced NLP applications like chatbots and intelligent data analysis systems.
Try NLTK with FlowHunt
Discover how NLTK can enhance your NLP projects. Build smart chatbots and AI tools using FlowHunt's intuitive platform.